Das Wetter in Hamburg ist immer schrecklich. Kalt, neblig, regnerisch. Echt jetzt?
Vor kurzem bin ich von Süddeutschland nach Hamburg, in den “Norden”, gezogen. Alle sagen, dass das Wetter dort schrecklich ist. Wirklich? Selbst die Hamburger beschweren sich immer über das Wetter. Aber um ehrlich zu sein, habe ich persönlich keine negativen Unterschiede gespürt. Ich hatte wirklich das Gefühl, dass das Wetter besser ist als in Bayern. Wenn ich meinen Kollegen von meinen persönlichen Vermutungen erzählt habe, haben sie immer gesagt: “Nein, da liegst du falsch. Das Wetter ist im Süden besser”.
Wir sollten Fakten schaffen, nicht glauben, dachte ich.
Ich beschloss, Wetterdaten von openweathermap.org aus verschiedenen Städten zu protokollieren. Ich habe Python verwendet, um json-Daten herunterzuladen und zu extrahieren. Die Daten werden dann in eine MariaDB-Datenbank eingefügt. Für die Visualisierung und Analyse der Daten verwende ich Metabase, um einige schöne Dashboards zu erstellen. So sieht das Design der Datenbank aus:
CREATE TABLE `weather_city` (
`id` int(11) NOT NULL,
`name` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE TABLE `weather_data` (
`id_city` int(11) NOT NULL,
`timestamp` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
`temp` double NOT NULL,
`pressure` double NOT NULL,
`humidity` double NOT NULL,
`windspeed` double NOT NULL,
`clouds` double NOT NULL,
`rain3h` double DEFAULT NULL,
`snow3h` int(11) DEFAULT NULL,
`sunrise` timestamp NULL DEFAULT NULL,
`sunset` timestamp NULL DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8
Jetzt kommt der nächste Teil, wie man die Daten von openweathermap.org mit einem Python-Skript extrahiert und in die Datenbank einfügt:
import sys
import requests
import MySQLdb
def get_weather(api_key, city_id):
url = "https://api.openweathermap.org/data/2.5/weather?id={}&units=metric&appid={}".format(city_id, api_key)
print url
r = requests.get(url)
return r.json()
def main():
conn = MySQLdb.connect(
user='USERNAME',
passwd='PASSWORD',
db='DATABASENAME',
host='HOSTNAME',
charset="utf8",
use_unicode=True)
cursor = conn.cursor()
api_key = "YOURAPIKEYFROMOPENWEATHER"
sql = "SELECT * FROM `weather_city`"
cursor.execute(sql)
cities = cursor.fetchall()
city_ids = []
for city in cities:
city_ids.append (city[0])
for city_id in city_ids:
weather = get_weather(api_key, city_id)
temp = weather["main"]["temp"]
humidity = weather["main"]["humidity"]
pressure = weather["main"]["pressure"]
windspeed = weather["wind"]["speed"]
clouds = weather["clouds"]["all"]
sunrise = weather["sys"]["sunrise"]
sunset = weather["sys"]["sunset"]
timestamp = weather["dt"]
sql = "INSERT INTO weather_data (id_city, timestamp, temp, pressure, humidity, windspeed, clouds, sunrise, sunset) VALUES (%s, FROM_UNIXTIME(%s), %s, %s, %s, %s, %s, FROM_UNIXTIME(%s), FROM_UNIXTIME(%s))"
val = (city_id, timestamp, temp, pressure, humidity, windspeed, clouds, sunrise, sunset)
cursor.execute(sql, val)
conn.commit()
if __name__ == '__main__':
main()
Und hier sind die Ergebnisse: In Hamburg ist es wärmer als in München, das 600 km südlicher liegt!
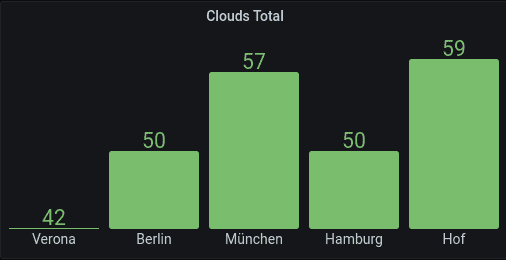
Und: In München ist es viel bewölkter als in Hamburg. Also, ich hatte Recht, der Rest ist nur Fake News :-)
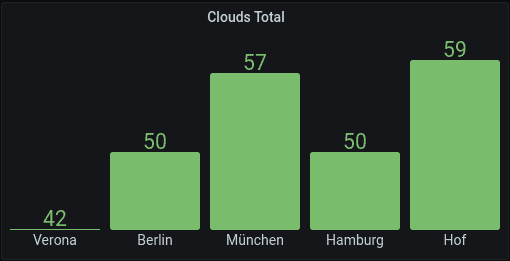
Leave a Reply